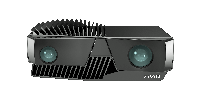 |
Zivid C++ API
1.8.1+6967bc1b-1
Defining the Future of 3D Machine Vision
|
Go to the documentation of this file.
63 class CloudVisualizer;
81 using StateCallback [[deprecated(
"1.2.0: No longer in use elsewhere")]] = std::function<void(CameraState)>;
230 std::shared_ptr<class CameraImpl> m_impl;
245 : m_settings{ camera.settings() }
255 : m_settings{ std::move(other.m_settings) }
256 , m_camera{ other.m_camera }
258 other.m_apply =
false;
271 template<
typename Setting>
274 m_settings.
set(value);
279 template<
typename Setting>
283 return std::move(setter);
287 bool m_apply{
true };
297 template<
typename Setting>
298 SettingsBatch
operator<<(Camera &camera, Setting value)
300 return SettingsBatch{ camera } << value;
ZIVID_API_EXPORT void stopLive()
Stop live (continuous) capturing of frames
SettingsBatch(Camera &camera)
Construct a SettingsBatch helper class on a camera
Definition: Camera.h:283
ZIVID_API_EXPORT CameraIntrinsics intrinsics() const
Get the intrinsic parameters of the camera
ZIVID_API_EXPORT bool operator==(const Camera &other) const
Check if two camera instances represent the same device
SettingsBatch & set(const Setting &value)
Cache the setting until object destruction
Definition: Camera.h:311
ZIVID_API_EXPORT Camera()
Constructor
~SettingsBatch() noexcept(false)
Apply all cached settings to the camera
Definition: Camera.h:301
ZIVID_API_EXPORT std::vector< uint8_t > userData() const
Read user data from camera
ZIVID_API_EXPORT void startLive()
Start live (continuous) capturing of frames
ZIVID_API_EXPORT size_t userDataMaxSizeBytes() const
Get the maximum number of bytes of user data that can be stored in the camera
ZIVID_API_EXPORT Settings settings() const
Get the current camera settings
ZIVID_API_EXPORT void setFrameCallback(FrameCallback callback)
Set a callback that is executed every time a new frame is ready
std::function< void(Frame)> FrameCallback
Callback for frames
Definition: Camera.h:117
ZIVID_API_EXPORT Frame2D capture2D(const Settings2D &settings)
Capture a single 2D frame
SettingsBatch & operator=(const SettingsBatch &)=delete
ZIVID_API_EXPORT void setSettings(Settings settings)
Update the camera settings
ZIVID_API_EXPORT std::string toString() const
Get string representation of the camera info
ZIVID_API_EXPORT CameraRevision revision() const
Get the camera revision
ZIVID_API_EXPORT Camera & operator=(const Camera &other) noexcept
Copy assignment
ZIVID_API_EXPORT bool operator!=(const Camera &other) const
Check if two camera instances represent different devices
ZIVID_API_EXPORT Camera & connect()
Connect to the camera
ZIVID_API_EXPORT std::string modelName() const
Get the model name
ZIVID_API_EXPORT void disconnect()
Disconnect from the camera and free all resources associated with it
ZIVID_API_EXPORT CameraState state() const
Get the current camera state
friend SettingsBatch operator<<(SettingsBatch &&setter, Setting value)
Cache the setting until object destruction
Definition: Camera.h:319
ZIVID_API_EXPORT ~Camera()
Destructor
void set(const std::string &fullPath, const std::string &value)
Set a value from string by specifying the path
ZIVID_API_EXPORT Frame capture()
Capture a single 3D frame
std::function< void(CameraState)> StateCallback
Callback for state
Definition: Camera.h:120
ZIVID_API_EXPORT void setComputeDevice(ComputeDevice device)
Connect the camera to a given Compute device
The main Zivid namespace. All Zivid code is found here
Definition: Application.h:52
ZIVID_API_EXPORT DeviceCloud allocateDeviceCloud()
Allocate a new point cloud on the Compute device
ZIVID_API_EXPORT ComputeDevice computeDevice()
Get Compute device
#define ZIVID_API_EXPORT
Definition: APIExport.h:56
ZIVID_API_EXPORT SerialNumber serialNumber() const
Get the serial number of the Zivid camera
ZIVID_API_EXPORT void writeUserData(const std::vector< uint8_t > &data)
Write user data to camera. The total number of writes supported depends on camera model and size of d...
ZIVID_API_EXPORT std::string firmwareVersion() const
Get the camera's firmware version
ZIVID_API_EXPORT std::ostream & operator<<(std::ostream &stream, const Camera &camera)
Serialize the value to a stream