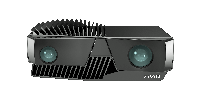 |
Zivid C++ API
1.8.1+6967bc1b-1
Defining the Future of 3D Machine Vision
|
ZIVID_API_EXPORT FrameInfo info() const
Get information collected at the time of the frame capture.
ZIVID_API_EXPORT PointCloud getPointCloud() const
Copies the point cloud to the CPU and returns it.
ZIVID_API_EXPORT DeviceCloud deviceCloud() const
Returns the point cloud on the Compute device.
ZIVID_API_EXPORT void save(const std::string &fileName) const
Save the frame to file
ZIVID_API_EXPORT std::string toString() const
Get string representation of the frame
ZIVID_API_EXPORT Frame()
Construct a new frame.
ZIVID_API_EXPORT void load(const std::string &fileName)
Load a frame from a Zivid data file.
The main Zivid namespace. All Zivid code is found here
Definition: Application.h:52
ZIVID_API_EXPORT CameraState state() const
Get the camera state data at the time of the frame capture.
#define ZIVID_API_EXPORT
Definition: APIExport.h:56
ZIVID_API_EXPORT Settings settings() const
Get the settings for the API at the time of the frame capture.