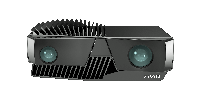 |
Zivid C++ API
1.8.1+6967bc1b-1
Defining the Future of 3D Machine Vision
|
Go to the documentation of this file.
77 x =
y =
z = std::numeric_limits<float>::quiet_NaN();
87 inline void setRgba(
unsigned char r,
unsigned char g,
unsigned char b,
unsigned char a)
89 rgba = static_cast<uint32_t>((a << 24) | (r << 16) | (g << 8) | b);
93 inline void setRgb(
unsigned char r,
unsigned char g,
unsigned char b)
111 inline unsigned char alpha()
const
113 return (
rgba >> 24) & 0xff;
117 inline unsigned char red()
const
119 return (
rgba >> 16) & 0xff;
123 inline unsigned char green()
const
125 return (
rgba >> 8) & 0xff;
129 inline unsigned char blue()
const
131 return (
rgba & 0xff);
137 unsigned short r =
red();
140 return static_cast<unsigned char>((r + g + b) / 3);
float contrast
Contrast.
Definition: Point.h:141
uint32_t rgba
Color (red,green,blue,alpha)
Definition: Point.h:142
unsigned char blue() const
Get the blue color value for the point
Definition: Point.h:208
unsigned char red() const
Get the red color value for the point
Definition: Point.h:196
void setIntensity(unsigned char val)
Set the intensity for the point (sets r,g and b to identical values)
Definition: Point.h:178
unsigned char green() const
Get the green color value for the point
Definition: Point.h:202
void setNaN()
Invalidate the point (set it to Not-a-Number)
Definition: Point.h:154
float y
Y coordinate.
Definition: Point.h:139
void setContrast(float value)
Set the contrast for the point
Definition: Point.h:184
Point()
Construct a 3D point at (0,0,0) with contrast 0 and color values (r,g,b,a) = (0,0,...
Definition: Point.h:145
unsigned char alpha() const
Get the alpha value for the point
Definition: Point.h:190
float x
X coordinate.
Definition: Point.h:138
void setRgba(unsigned char r, unsigned char g, unsigned char b, unsigned char a)
Set the r,g,b and alpha color value for the point
Definition: Point.h:166
unsigned char intensity() const
Get the intensity of the point
Definition: Point.h:214
bool isNaN() const
Check whether the point is valid (Not-a-Number)
Definition: Point.h:160
The main Zivid namespace. All Zivid code is found here
Definition: Application.h:52
void setRgb(unsigned char r, unsigned char g, unsigned char b)
Set the r,g,b color value for the point
Definition: Point.h:172
float z
Z coordinate.
Definition: Point.h:140